はじめに
JavaScriptを利用してAPIから値を取得し、画面へ反映する方法を共有するため、シンプルなWEBアプリを作ってみる会を行うこととした。
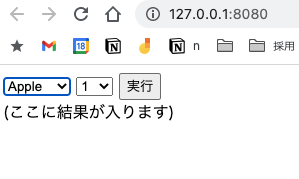
サーバを作る(with Node.js + express)
nodeの準備
$ npm init
nodeライブラリのインストール
$ npm install express body-parser cors
サーバのコード(app.js)を実装
const express = require('express');
const cors = require('cors');
const app = express();
const PORT = 4000; // ポート番号を4000に
app.use(express.json());
app.use(cors());
let items = [];
// Create
app.post('/items', (req, res) => {
const { name, price, description } = req.body;
const newItem = {
id: Date.now(),
name,
price,
description,
};
items.push(newItem);
res.status(201).json(newItem);
});
// Read
app.get('/items', (req, res) => {
res.json(items);
});
// Launch server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
サーバの起動
$ node app.js
起動した結果、いかが表示されたので、期待通り実装できたことが確認できた
$ node app.js
Server is running on port 4000
データを入れてみる
curl -X POST -H "Content-Type: application/json" -d '{"name": "Apple", "price": 100, "description": "apple"}' http://localhost:4000/items
curl -X POST -H "Content-Type: application/json" -d '{"name": "Banana", "price": 150, "description": "banana"}' http://localhost:4000/items
curl -X POST -H "Content-Type: application/json" -d '{"name": "Cherry", "price": 200, "description": "cherry"}' http://localhost:4000/items
データを見てみる
ブラウザでhttp://localhost:4000/itemsにアクセスしてみると、上記で登録した内容が表示される

WEBサイトを作る
下準備(nodeとwebサーバ起動用のモジュールをインストール)
$ cd front
$ npm init
$ npm install http-server
フロントエンドのコード(index.html)作成
「商品」と「個数」を入力したら、合計金額を表示する
商品の金額などの情報は、サーバから取得する
- 入力系
- プルダウン
- 商品選択用
- 個数選択用
- 実行ボタン
- プルダウン
- 出力系
- div(結果入力用)
- 処理
- 初期化(前準備)
- Itemクラスの作成
- Items変数の作成
- fetchを使ってサーバから値を取得する★
- fetchによるAPI呼び出し
- 商品選択用プルダウンの中身を作る
- 個数選択用プルダウンの中身を作る
- 実行ボタンの動作を作る
- 「商品選択用」と「個数選択用」プルダウンから入力値を取得
- 取得内容から商品の価格 x 個数を計算
- 計算結果を画面へ表示
- 初期化(前準備)
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<!-- 商品選択用プルダウン -->
<select id="name"></select>
<!-- 個数選択用プルダウン -->
<select id="count"></select>
<!-- 実行ボタン -->
<input id="calculate" type="button" value="実行">
<!-- 結果入れ -->
<div id="result">(ここに結果が入ります)</div>
<script>
// 初期化(前準備)
class Item {
constructor(name,price,description){
this.name = name;
this.price = price;
this.description = description;
}
}
let Items = [];
// fetchを使ってサーバから値を取得する★
fetch('http://localhost:4000/items')
.then(response => response.json())
.then(data => {
// サーバから取得した値をItemsに入れる
data.forEach(item => {
Items.push(new Item(item.name, item.price, item.description));
// 商品選択用プルダウンの中身を作る
const option = document.createElement('option');
option.textContent = item.name;
option.value = item.name;
document.getElementById('name').appendChild(option);
})
})
// 個数選択用プルダウンの中身を作る
for (let i = 1; i <= 100; i += 10) {
const option = document.createElement('option');
option.textContent = i;
option.value = i;
document.getElementById('count').appendChild(option);
}
// 実行ボタンの動作を作る
document.getElementById('calculate').addEventListener('click', () => {
const selectedItemName = document.getElementById('name').value;
const selectedItem = Items.find(item => item.name === selectedItemName);
const count = parseInt(document.getElementById('count').value, 10);
const result = selectedItem.price * count;
document.getElementById('result').textContent = `Total price: ${result}`;
});
</script>
</body>
</html>