はじめに
本投稿で書くAlexa道場の会は以下
- Episode 12 コーヒーショップのコードを完成せよう
前回、「何がわからないのか分からない状態」になったので、もう一回ビデオを見て、ビデオで達成しようとしていたことができるかどうかリトライすることにした
何が分かったのかまとめてみた
コーヒーショップをのスキルを完成させるためには、ここまで設定した内容に加えて、いくつか追加・変更が必要となる。
- LaunchRequestHandler
- LaunchRequestHandlerとは、「コーヒーショップを開いて」などで、スキルが起動した場合で、かつ、その他に何も入力がない場合(「
- コーヒーショップを開いて コーヒーを注文して」みたいに)の処理を書くメソッド
- ここが、デフォルトだと、「Welcome, you can say Hello or ・・・」のように意味が分からない文章になっているので、コーヒーショップスキルとして、あるべき文言に置き換える
- 「コーヒーショップへようこそ。今日は何にしますか?」のような文言にする。
- 8秒立っても応答がない場合に対応するために、repromptというメソッドを利用して、8秒立ったら追加で発話するようにする
- ここが、デフォルトだと、「Welcome, you can say Hello or ・・・」のように意味が分からない文章になっているので、コーヒーショップスキルとして、あるべき文言に置き換える
例
const LaunchRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'LaunchRequest';
},
handle(handlerInput) {
console.log(`1111111111111111111`);
const speakOutput = '111コーヒーショップへようこそ。今日は何にしますか?';
const reprompt = '8秒たったぞ。早く注文しろよ。いらねえのか?'
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(reprompt)
.getResponse();
}
};
しかし、repromptが期待通り動かない。ここは別途追求してみる。一旦飛ばす。
OrderIntentHandler
- OrderIntentHandlerとは、「コーヒーを注文して」などが入った場合の処理を定義するためのメソッド
- ここが、デフォルトだと、「Hello World」のように意味が分からない文章になっているので、コーヒーショップスキルとして、あるべき文言に置き換える
- 「コーヒーショップへようこそ。今日は何にしますか?」のような文言にする。
例
const OrderIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'OrderIntent';
},
handle(handlerInput) {
const speakOutput = 'コーヒーですね。ありがとうございます。';
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
ちなみに、ここはrepromptは必要ない。
HelpIntentHandler
- HelpIntentHandlerとは、「ヘルプ」と言われた場合の処理を定義するメソッド
- なお、このインテントには、制約がある
- 使い方を教えた後に、問いかけで終わる必要がある
- LaunchRequestHandlerと同じ内容で構わない
例
const HelpIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.HelpIntent';
},
handle(handlerInput) {
const speakOutput = 'ヘルプ?コーヒーショップへようこそ。今日は何にしますか?';
const reprompt = 'ヘルプ言ってから8秒たったぞ。早く注文しろよ。いらねえのか?'
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
ちなみに、こっちもrepromptが動かない
CancelAndStopIntentHandler
- CancelAndStopIntentHandlerとは、「キャンセル」と言われた場合の処理を定義するメソッド
- ここが、デフォルトだと、「GoodBye!」のようにちょっと雑な文章になっているので、コーヒーショップスキルとして、あるべき文言に置き換える
- 「またね」のような文言にする。
例
const CancelAndStopIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& (Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.CancelIntent'
|| Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.StopIntent');
},
handle(handlerInput) {
const speakOutput = 'またね!';
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
SessionEndedRequestHandler
- SessionEndedRequestHandlerは、スキルが終了したときに実行される処理を定義するメソッド
- 特に喋らせることは必要ないので、デフォルトのままで問題ない
例
const SessionEndedRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'SessionEndedRequest';
},
handle(handlerInput) {
console.log(`~~~~ Session ended: ${JSON.stringify(handlerInput.requestEnvelope)}`);
// Any cleanup logic goes here.
return handlerInput.responseBuilder.getResponse(); // notice we send an empty response
}
};
IntentReflectorHandler
- IntentReflectorHandlerとは、指定したインテントがない場合に、実行される処理を定義するメソッド
- ここが、デフォルトだと、「You just triggerd ・・・」のように英語でわかりにくいので、コーヒーショップスキルとして、あるべき文言に置き換える
- 「〜というインテントが呼ばれました」のような文言にする。
しかし、期待通り動かない。
コーヒー以外の注文をした場合に、このハンドラが呼ばれる想定であったが、実際にはコーヒー以外を注文すると、別なハンドラが実行されてしまう。
具体的には、我々が独自に追加してみたunkoIntentHandlerが呼ばれてしまう
【unkoIntentHandler】
const unkoIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'unkoIntent';
},
handle(handlerInput) {
return handlerInput.responseBuilder
.speak("うんこですね。")
//.reprompt('add a reprompt if you want to keep the session open for the user to respond')
.withSimpleCard("いやー","すごい")
.speak("まさしく。")
.speak("うんこですねー!!。")
.withSimpleCard("はは","はー")
.getResponse();
}
}
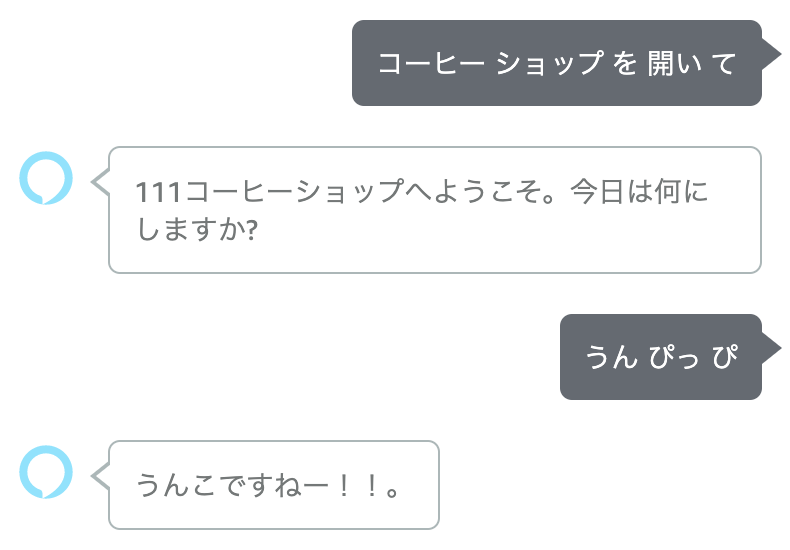
ここは別途追求してみる。一旦飛ばす。
ErrorHandler
- ErrorHandlerとは、エラーが起きたときに実行される処理を定義するメソッドである
- ここが、デフォルトだと、「You just triggerd ・・・」のように英語でわかりにくいので、コーヒーショップスキルとして、あるべき文言に置き換える。
例
const ErrorHandler = {
canHandle() {
return true;
},
handle(handlerInput, error) {
const speakOutput = 'エラーです';
console.log(`~~~~ Error handled: ${JSON.stringify(error)}`);
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
おわりに
- ひとまず、前回起きていた状況を一旦整理した
- 今回は1人休みだったのもあり、続きはまた次回にする。